Introduction
In this blog, I will share how we can increase code coverage for unit tests by using Coverage.py.
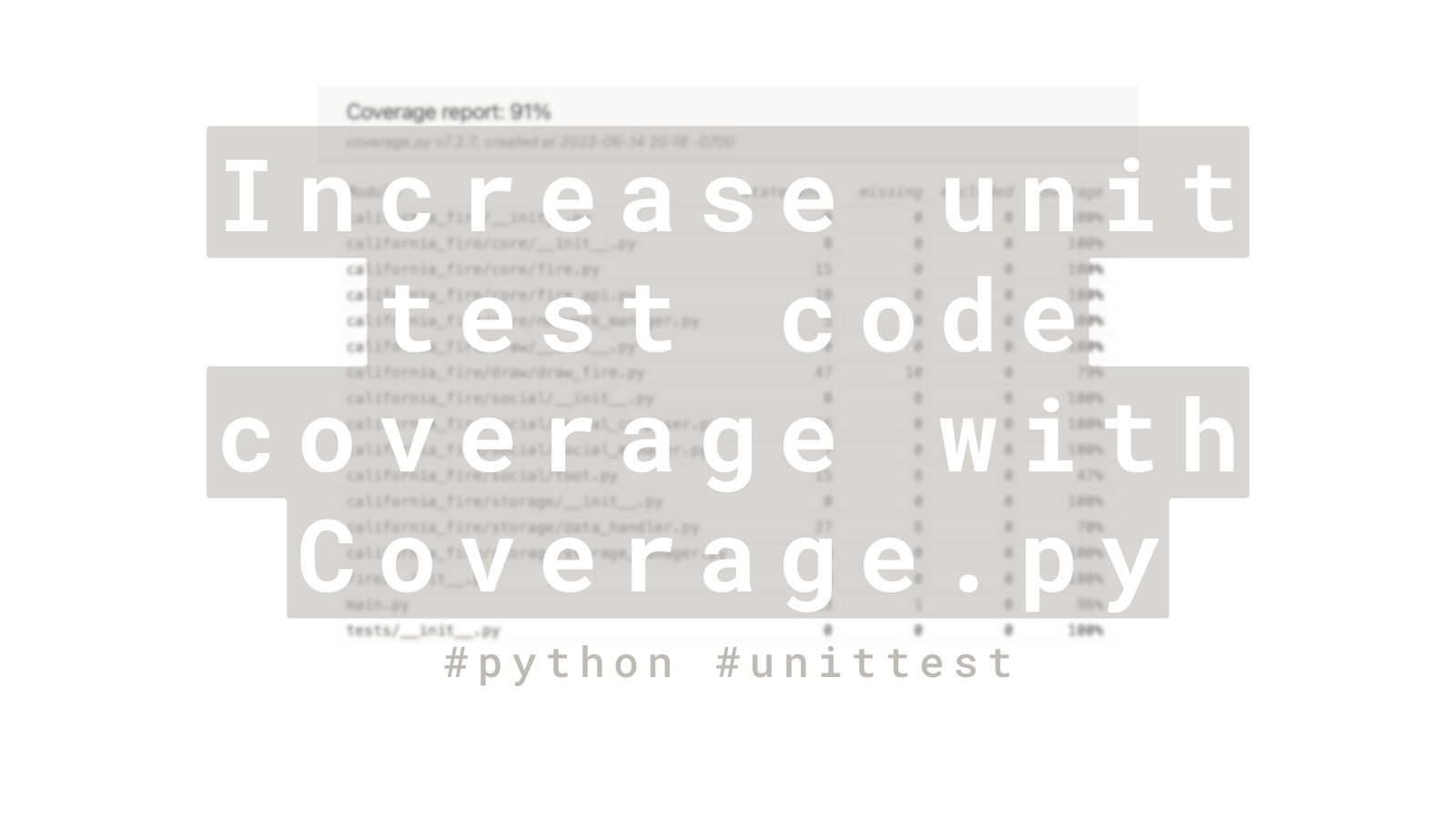
Unit testing is at the lower level of the Test Pyramid, where it can provide faster results and greater isolation. This helps us obtain faster feedback and prevents unexpected behavior in our application.
Therefore, we need a tool to help identify areas that have not been tested, and Coverage.py is very easy to use.
Let’s first install Coverage.
python3 -m pip install coverage
In my project, I’m using the unittest framework for unit testing.
python -m unittest #To run the tests
To run test suite.
(california_fire) ➜ california_fire git:(main) ✗ coverage run -m unittest discover
Year: 2023
......................No fire fire from api.
.No new fire.
..
----------------------------------------------------------------------
Ran 25 tests in 0.050s
OK
Then, create report.
(california_fire) ➜ california_fire git:(main) ✗ coverage report
Name Stmts Miss Cover
----------------------------------------------------------------
california_fire/__init__.py 0 0 100%
california_fire/core/__init__.py 0 0 100%
california_fire/core/fire.py 15 0 100%
california_fire/core/fire_api.py 10 0 100%
california_fire/core/network_manager.py 5 0 100%
california_fire/draw/__init__.py 0 0 100%
california_fire/draw/draw_fire.py 47 10 79%
california_fire/social/__init__.py 0 0 100%
california_fire/social/social_composer.py 16 0 100%
california_fire/social/social_manager.py 7 0 100%
california_fire/social/toot.py 15 8 47%
california_fire/storage/__init__.py 0 0 100%
california_fire/storage/data_handler.py 27 8 70%
california_fire/storage/storage_manager.py 8 0 100%
fire/__init__.py 0 0 100%
main.py 23 1 96%
tests/__init__.py 0 0 100%
tests/core/__init__.py 0 0 100%
tests/core/test_api.py 13 1 92%
tests/core/test_network_manager.py 24 1 96%
tests/draw/__init__.py 0 0 100%
tests/draw/test_draw_fire.py 39 1 97%
tests/social/__init__.py 0 0 100%
tests/social/test_social_composer.py 34 1 97%
tests/social/test_social_manager.py 18 1 94%
tests/storage/__init__.py 0 0 100%
tests/storage/test_data_handler.py 42 1 98%
tests/storage/test_storage_manager.py 24 1 96%
tests/test_main.py 40 1 98%
utilities/__init__.py 0 0 100%
----------------------------------------------------------------
TOTAL 407 35 91%
Create html report.
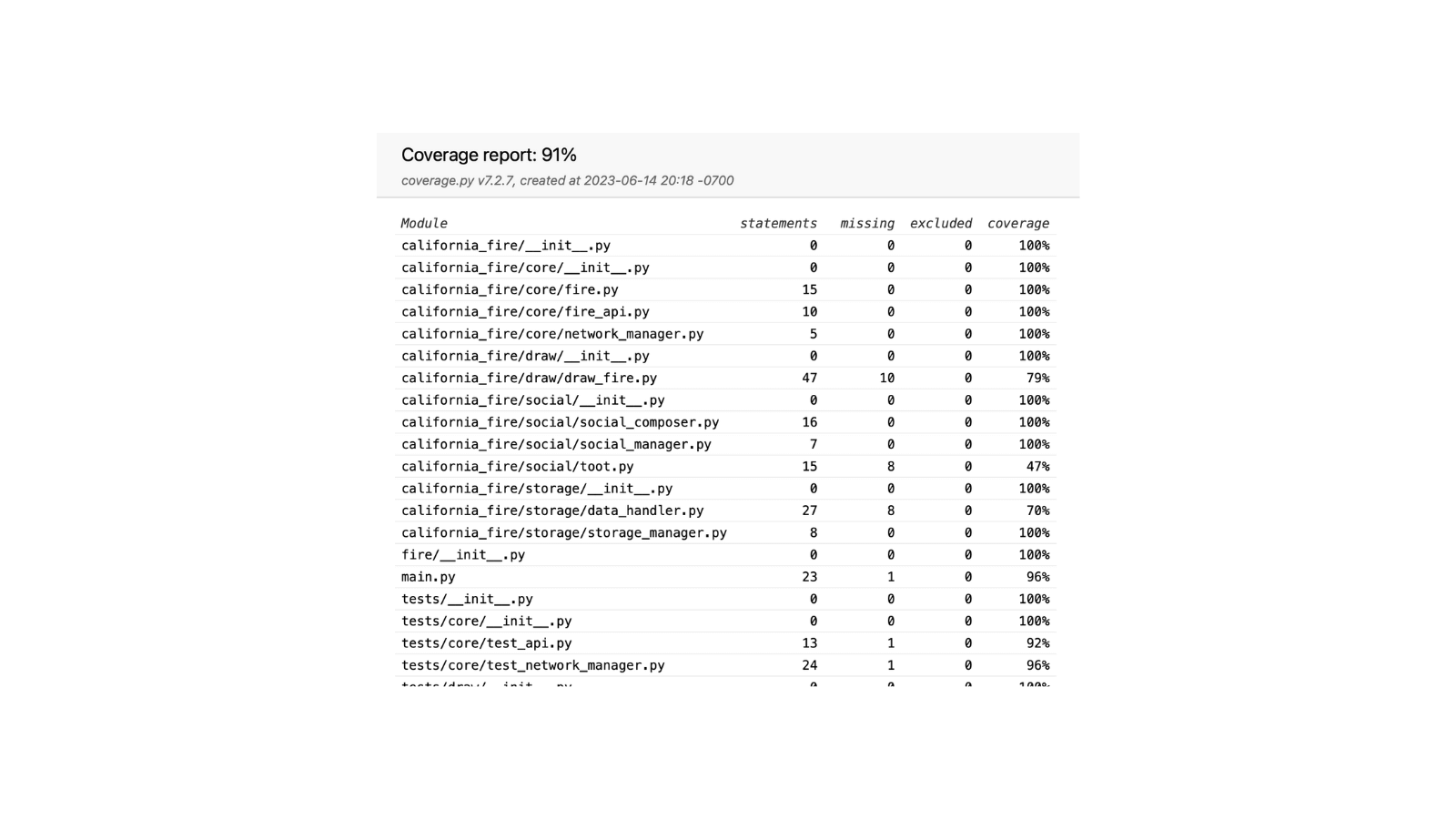
You can click to view the details for each result.
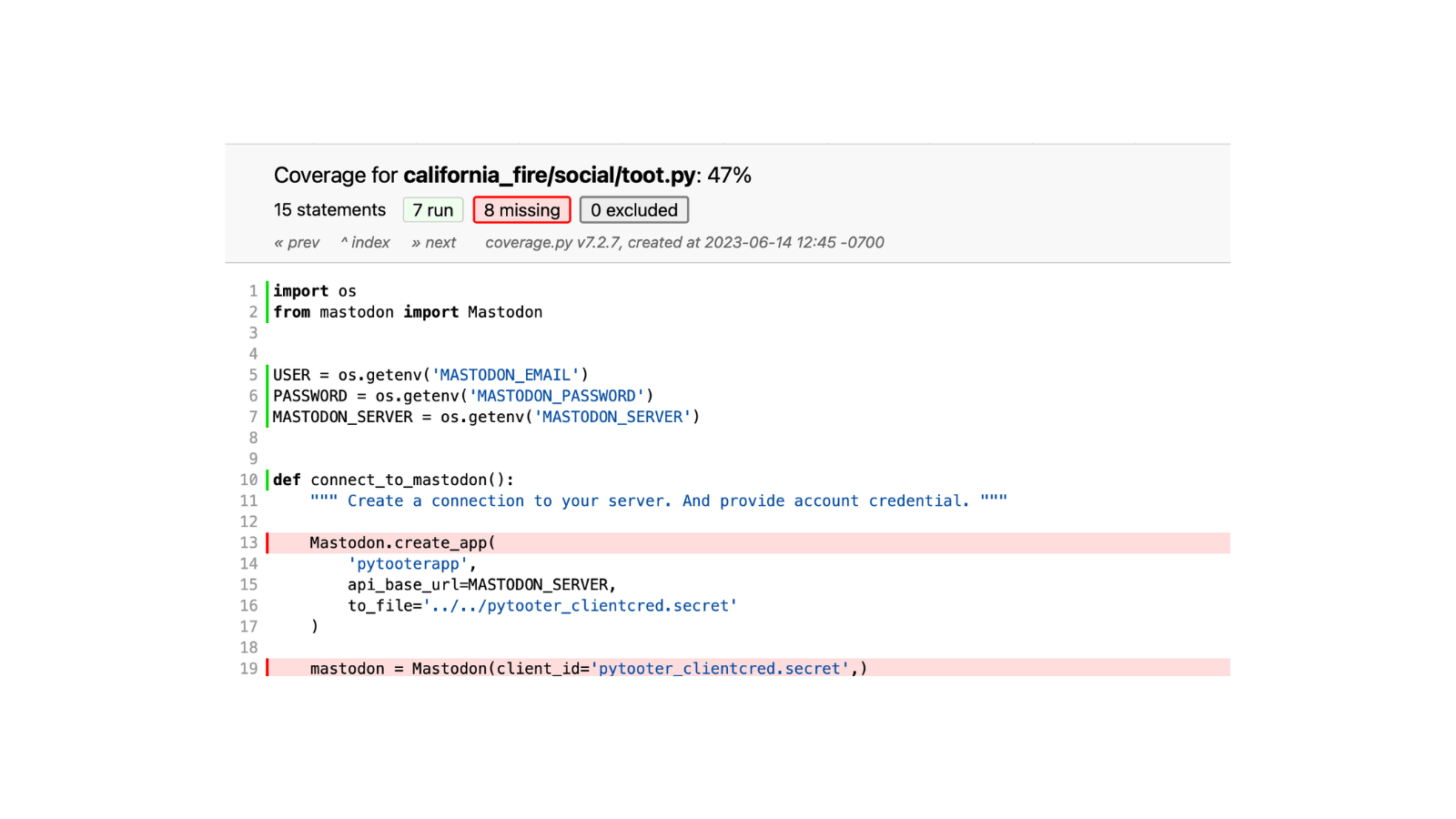
This will help you identify areas that have not been tested yet. You can then proceed to write tests for those areas.
In my project, I added more tests to cover those areas, and as a result, my code coverage increased.
(california_fire) ➜ california_fire git:(main) ✗ coverage report
Name Stmts Miss Cover
----------------------------------------------------------------
california_fire/__init__.py 0 0 100%
california_fire/core/__init__.py 0 0 100%
california_fire/core/fire.py 15 0 100%
california_fire/core/fire_api.py 10 0 100%
california_fire/core/network_manager.py 5 0 100%
california_fire/draw/__init__.py 0 0 100%
california_fire/draw/draw_fire.py 47 0 100%
california_fire/social/__init__.py 0 0 100%
california_fire/social/social_composer.py 16 0 100%
california_fire/social/social_manager.py 7 0 100%
california_fire/social/toot.py 15 0 100%
california_fire/storage/__init__.py 0 0 100%
california_fire/storage/data_handler.py 27 0 100%
california_fire/storage/storage_manager.py 8 0 100%
main.py 23 1 96%
tests/__init__.py 0 0 100%
tests/core/__init__.py 0 0 100%
tests/core/test_api.py 13 1 92%
tests/core/test_network_manager.py 24 1 96%
tests/draw/__init__.py 0 0 100%
tests/draw/test_draw_fire.py 67 1 99%
tests/social/__init__.py 0 0 100%
tests/social/test_social_composer.py 34 1 97%
tests/social/test_social_manager.py 18 1 94%
tests/social/test_toot.py 18 1 94%
tests/storage/__init__.py 0 0 100%
tests/storage/test_data_handler.py 62 1 98%
tests/storage/test_storage_manager.py 24 1 96%
tests/test_main.py 53 1 98%
----------------------------------------------------------------
TOTAL 486 10 98%
If you’re interested in learning more, please visit the Coverage.py.
I would love to share more about how we can improve the quality of our application.
Stay tuned for more upcoming blog posts!